The Retool Embed Software Development Kit (SDK) is a powerful tool that allows developers to integrate Retool applications into their existing web applications easily.
This SDK provides the necessary components and APIs to embed Retool's low-code, customizable applications directly into web pages, enhancing the functionality and user experience without requiring extensive development work.
By using Retool Embed, businesses can leverage the full capabilities of Retool within their current web infrastructure, ensuring a cohesive and efficient workflow.
This article will give you a comprehensive overview of the details needed to use the retool embed function.
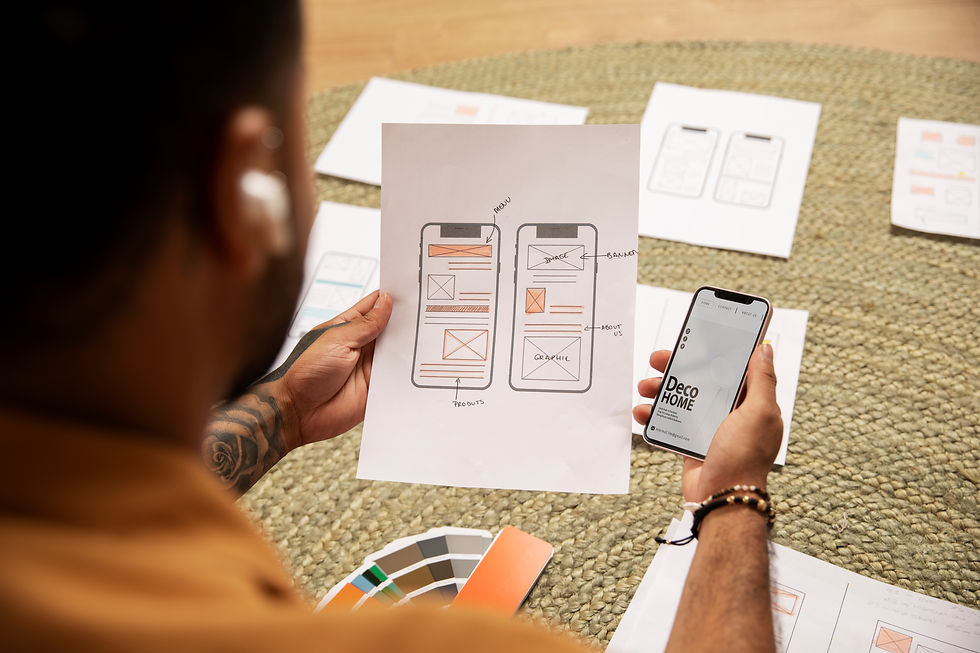
How to Embed a Retool App Using createRetoolEmbed Function
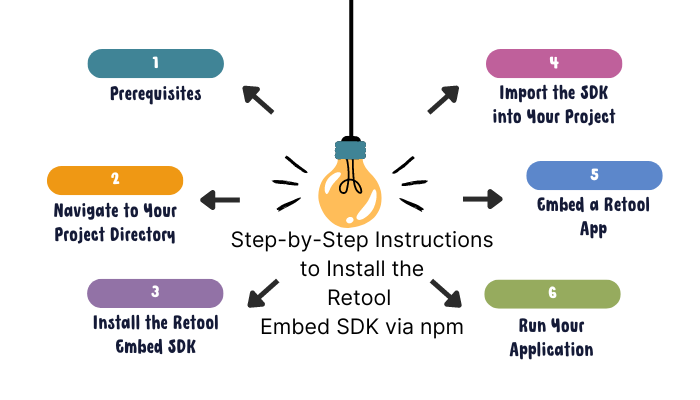
To embed Retool applications into your existing web application, you need to install the Retool Embed SDK.
The createRetoolEmbed function lets you easily embed Retool apps into your existing web applications. Here’s a step-by-step guide on how to use this function:
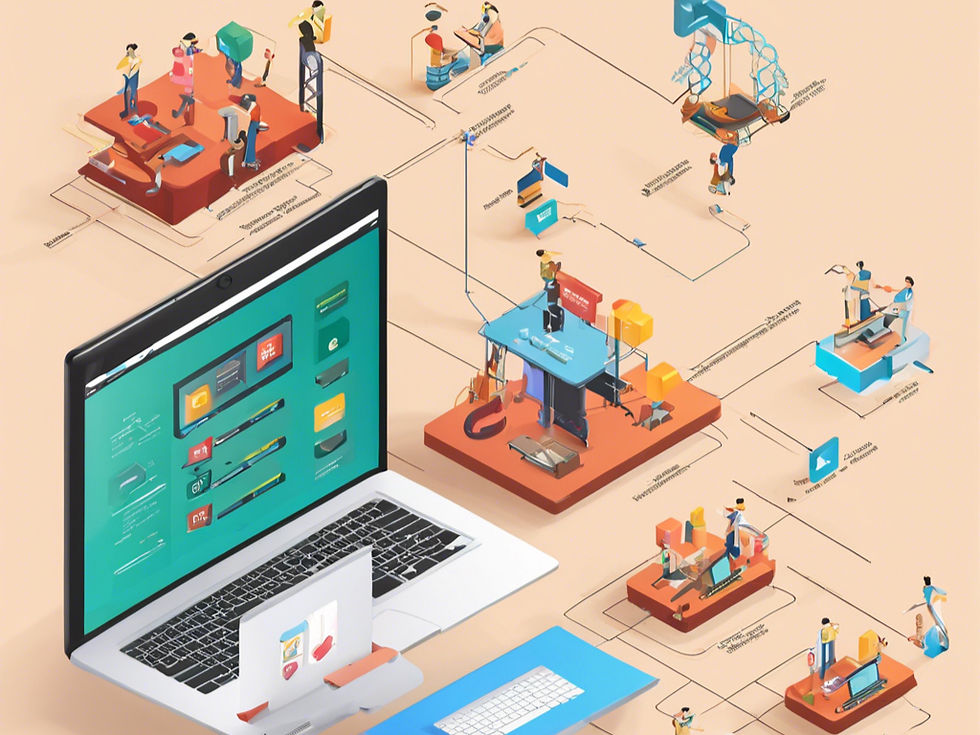
a) Prerequisites: Ensure you have Node.js and NPM installed on your machine. You can download and install Node.js from nodejs.org, which includes npm.
b) Navigate to Your Project Directory: Open your terminal or command prompt and navigate to the root directory of your web application project.
cd path/to/your/project
c) Install the Retool Embed SDK: Use npm to install the Retool Embed SDK by running the following command:
npm install @retool/embed
d) Import and Use the createRetoolEmbed Function: Import the createRetoolEmbed function into your JavaScript or TypeScript file and use it to embed your Retool app. Here’s an example:
import { createRetoolEmbed } from '@retool/embed';
// Create a container element for the embedded app
const container = document.getElementById('retool-embed-container');
// Configuration options
const config = {
style: {
width: '100%',
height: '600px',
},
data: {
key1: 'value1',
key2: 'value2',
},
onData: (data) => {
console.log('Data received from Retool app:', data);
},
};
// Embed the Retool app
createRetoolEmbed(container, config);
e) Add the Container Element to Your HTML: Ensure that the container element (retool-embed-container in this case) is present in your HTML:
<div id="retool-embed-container"></div>
Configuration Options Available: src, style, data, and onData
The createRetoolEmbed function provides several configuration options to customize the embedded Retool app:
src: The URL of the Retool app you want to embed.
style: An object specifying CSS styles to apply to the iframe containing the Retool app. Common styles include width and height.
style: {
width: '100%',
height: '600px',
}
data: Pass an object containing data to the Retool app. It can be used to prepopulate fields or configure the app dynamically.
data: {
key1: 'value1',
key2: 'value2',
}
onData: A callback function that receives data from the Retool app. This function is triggered when the app returns data to the host application.
onData: (data) => {
console.log('Data received from Retool app:', data);
}
Embedding Retool apps into your existing web applications using the createRetoolEmbed function is straightforward and provides extensive configuration options.
Creating an Authenticated Embed URL: Detailed Procedure and Code Snippets
To embed Retool apps that require authentication, you must create an authenticated embed URL. It involves generating a secure URL with appropriate tokens.
Step-by-Step Guide:
a) Generate an API Key:
Go to your Retool account settings and generate an API key.
b) Create an Authenticated Embed URL: Use the API key to create a secure, authenticated URL. Here’s a code snippet for generating an authenticated URL in Node.js:
const jwt = require('jsonwebtoken');
const payload = {
// Include any user-specific data or permissions
user: {
id: 'user-id',
email: 'user@example.com',
},
// Retool app-specific details
app: 'YourAppName',
};
// Secret key from Retool settings
const secret = 'your-retool-secret-key';
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
const embedUrl = `https://your-subdomain.retool.com/embed/${payload.app}?token=${token}`;
console.log('Authenticated Embed URL:', embedUrl);
c) Embed the Authenticated Retool App: Use the authenticated URL in your web application:
import { createRetoolEmbed } from '@retool/embed';
// Create a container element for the embedded app
const container = document.getElementById('retool-embed-container');
// Configuration options
const config = {
style: {
width: '100%',
height: '600px',
},
};
// Embed the Retool app
createRetoolEmbed(container, config);
d) Add the Container Element to Your HTML: Ensure that the container element (retool-embed-container in this case) is present in your HTML:
<div id="retool-embed-container"></div>
You can integrate powerful Retool functionalities into your existing infrastructure by following the steps and using the provided code snippets.
Purpose of Embedding a Public Retool App
Embedding a public Retool app into your existing application can serve multiple purposes, significantly enhancing the functionality and user experience of your application. Here are the main purposes:
1. Streamlined Operations
Unified Interface: Embedding a Retool app allows users to access various internal tools directly within their primary application, streamlining workflows and reducing the need to switch between different platforms.
Example: A CRM system with an embedded Retool app can provide sales teams with real-time data analytics and customer insights without leaving the CRM interface.
2. Enhanced Data Integration
Centralized Data Access: Retool can connect to multiple data sources, such as databases, APIs, and third-party services. Embedding these apps provides centralized access to all relevant data within your main application.
Seamless Data Operations: Users can perform data operations such as querying, updating, and reporting directly from the embedded app, enhancing efficiency.
3. Custom Internal Tools
Tailored Solutions: Retool allows the creation of custom internal tools tailored to specific business needs. Embedding these tools in your application ensures that users have access to functionalities designed specifically for their tasks.
Example: An embedded inventory management tool within an e-commerce platform can help manage stock levels, track orders, and generate reports.
4. Improved User Experience
Ease of Use: Embedding Retool apps provides a consistent user experience by integrating necessary tools and data within a single application, reducing the learning curve and improving user satisfaction.
Example: Customer support teams can access all the tools they need to resolve issues quickly within the main application, leading to faster response times and higher customer satisfaction.
5. Real-Time Updates and Collaboration
Live Data Access: Embedded Retool apps can provide real-time data access, ensuring that users are always working with the most up-to-date information.
Collaboration: Features such as shared dashboards and collaborative tools can be embedded to facilitate teamwork and improve decision-making processes.
You can explore some of the advanced embedding features from the upcoming section and learn how to use them practically.
Advanced Embedding Features
You can expand your knowledge on how to use the advanced Embedding features in your Retool by following the detailed steps mentioned in this section.
Two-Way Communication Between Retool and the Embedding Application
Enabling two-way communication between Retool and the embedding application enhances interactivity and integration, providing a seamless user experience. This is achieved through the use of the postMessage API, which facilitates secure and efficient message exchange between the parent webpage and the embedded Retool app.
How to Implement Two-Way Communication: Step-by-Step Setup Guide with Code Snippets
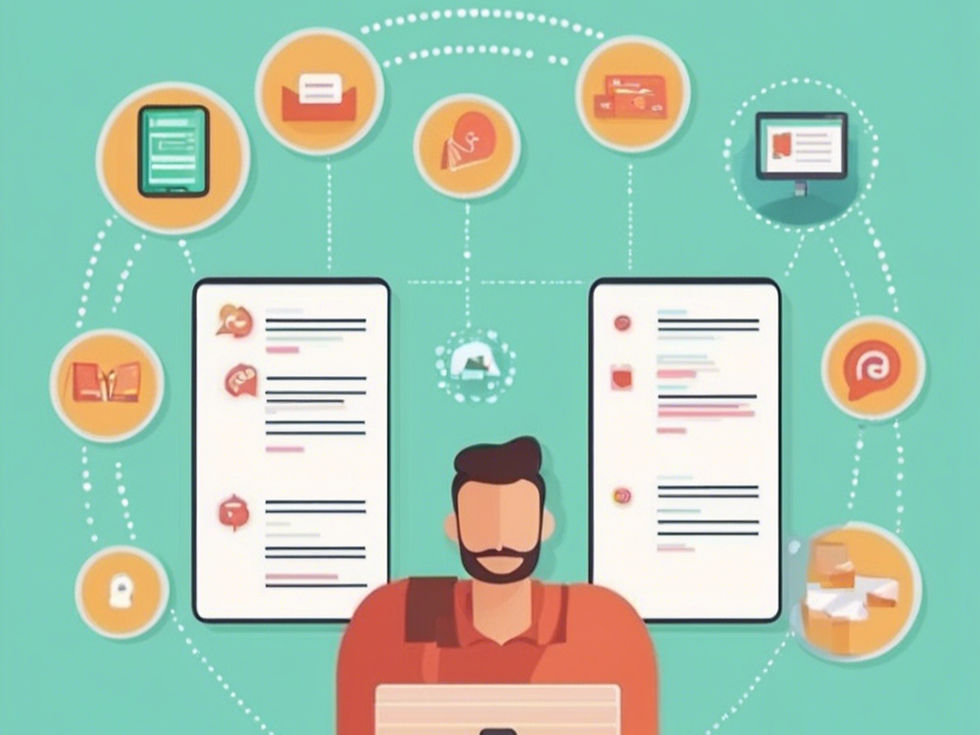
a) Set Up the Retool Embed: Make sure to embed the Retool app using the createRetoolEmbed function and set it up to handle incoming messages from the parent application.
import { createRetoolEmbed } from '@retool/embed';
// Create a container element for the embedded app
const container = document.getElementById('retool-embed-container');
// Configuration options
const config = {
style: {
width: '100%',
height: '600px',
},
// Optional: Define initial data to pass to the Retool app
data: {
initialKey: 'initialValue',
},
// Handle data sent from Retool
onData: (data) => {
console.log('Data received from Retool app:', data);
// Process the data received from the Retool app
},
};
// Embed the Retool app
const retoolEmbed = createRetoolEmbed(container, config);
b) Enable Message Handling in Retool: In your Retool app, use the window.postMessage API to communicate with the parent application. Add the following JavaScript code to your Retool app:
// Send a message to the parent application
window.parent.postMessage({ type: 'FROM_RETOOL', data: 'Hello from Retool' }, '*');
// Handle messages from the parent application
window.addEventListener('message', (event) => {
if (event.data.type === 'FROM_PARENT') {
console.log('Message from parent:', event.data);
// Process the message from the parent application
}
});
c) Send Messages from the Parent Application to Retool: In the parent application, use the postMessage method to send messages to the embedded Retool app:
// Reference to the embedded Retool iframe
const retoolIframe = document.querySelector('iframe');
// Send a message to the embedded Retool app
retoolIframe.contentWindow.postMessage({ type: 'FROM_PARENT', data: 'Hello from Parent' }, '*');
d) Handling Data Between Applications: Ensure that your parent application and Retool app are set up to handle and process the messages sent and received.
Benefits of Two-Way Communication
Real-Time Data Sync: Ensures that both the Retool app and the parent application can exchange data in real-time, critical for applications requiring immediate updates.
Enhanced User Interactions: Allows the parent application to react dynamically to changes or inputs within the Retool app, and vice versa, enhancing the responsiveness and interactive nature of your application.
Customizable Workflows: Enables complex, customized workflows where actions in one application can trigger responses in the other, tailored specifically to business needs.
Security Considerations
When implementing postMessage, it's crucial to ensure that the messages are secure:
Validate Message Origin: Always check the origin of incoming messages to ensure they are from trusted sources.
Secure Data Handling: Be cautious with the data received through messages. Validate and sanitize all inputs to avoid security vulnerabilities.
By setting up two-way communication between Retool and your embedding application, you can create a highly interactive and dynamic user experience.
The postMessage API facilitates this interaction, enabling both the parent application and the embedded Retool app to send and receive messages. For expert insights and tools to optimize your Retool applications, partner with ToolPioneers.
Now, let’s focus on generating secure embed URLs to ensure that only authorized users can access your Retool applications.
Generating an Embed URL
Generating an embed URL in Retool allows you to integrate Retool apps directly into your existing applications, providing seamless access to your internal tools and dashboards.
Best Practices for Securely Generating an Embed URL on the Backend
Generating an embed URL ensures that only authorized users can access your embedded Retool apps. Here are the best practices for securely generating an embed URL on the backend:
1. Use JWT for Secure Authentication:
JSON Web Tokens (JWT) is a secure way to transmit information between parties as a JSON object. JWT can be used to encode user information and permissions.
2. Include User-Specific Claims:
Add claims to the JWT payload that specify user-specific information, such as user ID, email, and roles. It ensures that the embedded app can identify and authorize the user.
3. Set Token Expiry:
Ensure that the JWT has an expiration time (exp claim) to limit the validity period—this helps prevent token misuse.
4. Sign the JWT Securely:
Sign the JWT with a strong secret key or an RSA private key. It ensures that the token is tamper-proof.
5. Generate the Embed URL:
Append the generated JWT to the Retool embed URL. This URL will embed the Retool app in your web application.
Example Code for Generating an Embed URL in Node.js:
const jwt = require('jsonwebtoken');
// User-specific payload
const payload = {
user: {
id: 'user-id',
email: 'user@example.com',
roles: ['admin', 'editor'],
},
app: 'YourAppName',
};
// Secret key from Retool settings
const secret = 'your-retool-secret-key';
// Generate JWT
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
// Generate the embed URL
const embedUrl = `https://your-subdomain.retool.com/embed/${payload.app}?token=${token}`;
console.log('Authenticated Embed URL:', embedUrl);
1. Backend Endpoint to Serve the Embed URL:
Create a backend endpoint for securely generating and serving the embed URL while ensuring the token generation logic is not exposed to the client.
Example Express.js Endpoint:
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
app.get('/generate-embed-url', (req, res) => {
// User-specific payload
const payload = {
user: {
id: 'user-id',
email: 'user@example.com',
roles: ['admin', 'editor'],
},
app: 'YourAppName',
};
// Secret key from Retool settings
const secret = 'your-retool-secret-key';
// Generate JWT
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
// Generate the embed URL
const embedUrl = `https://your-subdomain.retool.com/embed/${payload.app}?token=${token}`;
res.json({ embedUrl });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Embedding Retool apps into existing web applications has its own set of benefits, making it an essential part of working with applications in today’s technologically advanced world. Let’s discuss the benefits and advanced authentication options for embedding Retool apps into your existing web applications.
Benefits of Embedding Retool Apps into Existing Web Applications
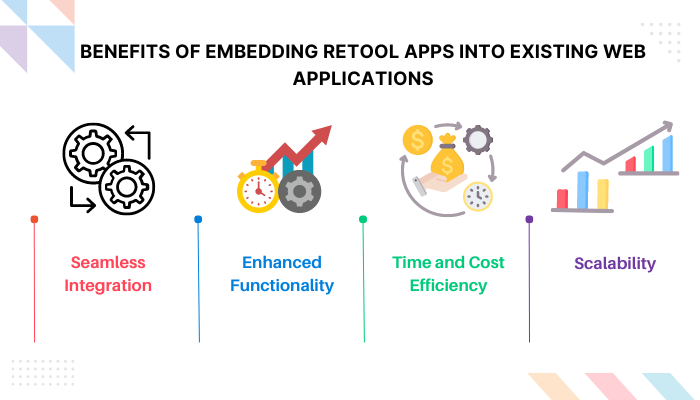
Seamless Integration: Embedding Retool apps ensures a consistent user experience by directly integrating advanced functionalities into your web applications. Users can interact with Retool features without leaving the main application environment.
Enhanced Functionality: Retool’s robust app-building platform allows for creating complex, data-driven interfaces. By embedding these apps, you can improve your web applications with powerful tools and capabilities tailored to your business needs.
Time and Cost Efficiency: Embedding Retool apps reduces the need to develop custom solutions from scratch. Retool’s low-code platform accelerates development, allowing quicker deployment and lower development costs.
Scalability: Retool apps can quickly scale with your business. As your needs evolve, you can update and expand the embedded Retool applications without major overhauls to your main web application.
Understanding Authentication Options for Retool Applications
Retool provides several authentication options to ensure secure access to embedded applications:
API Key Authentication: Use API keys to authenticate requests to Retool applications. This method is straightforward to implement, making it suitable for internal applications.
OAuth2: For a more secure and scalable solution, Retool supports OAuth2 authentication. This method allows you to integrate with various identity providers, ensuring only authorized users can access the embedded Retool apps.
SSO (Single Sign-On): Retool can integrate with your existing SSO solution, providing users with a seamless and secure login experience. It is beneficial for enterprise environments where centralized authentication is crucial.
JWT (JSON Web Tokens): Retool supports JWT authentication, enabling you to pass user identity information to the embedded applications securely. This method provides flexibility and security, ensuring that user data is protected.
Reference to the Documentation for Detailed Guidance
For detailed guidance on securely generating embed URLs and integrating Retool into your applications., refer to the official Retool documentation:
Following best practices for securely generating an embed URL ensures that your embedded Retool apps are accessible only to authorized users. By using JWT for authentication and including user-specific claims, you can enhance the security of your embedded applications.
For expert insight and guidance, feel free to contact Toolpioneers.
Refer to the Retool Embed documentation for more detailed instructions and advanced configurations.
Also, check out How can Toolpioneers provide Retool Support for Retool Developers.
Embedding Retool apps into your existing applications can provide several significant advantages, enhancing the functionality, efficiency, and overall user experience. You can follow up on the following section on “createRetoolEmbed” Retool embed function in more detail.