Implementing a practical search functionality for table filtering in Retool can significantly enhance user experience by allowing users to quickly and easily find specific data within large datasets.
However, achieving efficient table filtering, especially with large datasets, presents certain challenges. These challenges can be addressed by leveraging front-end and backend filtering techniques to ensure responsive and accurate search results, ultimately rewarding users with a seamless and efficient data search experience.
This article provides complete guidance on Retool search input. You can follow it and apply it to your application development process.
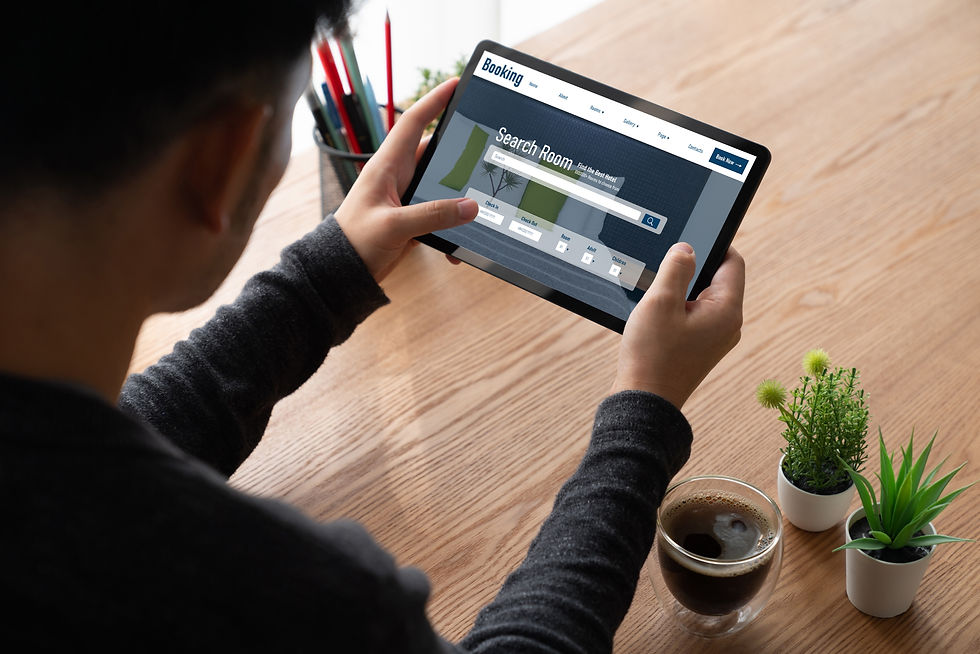
Why Is Retool Search Input Essential?
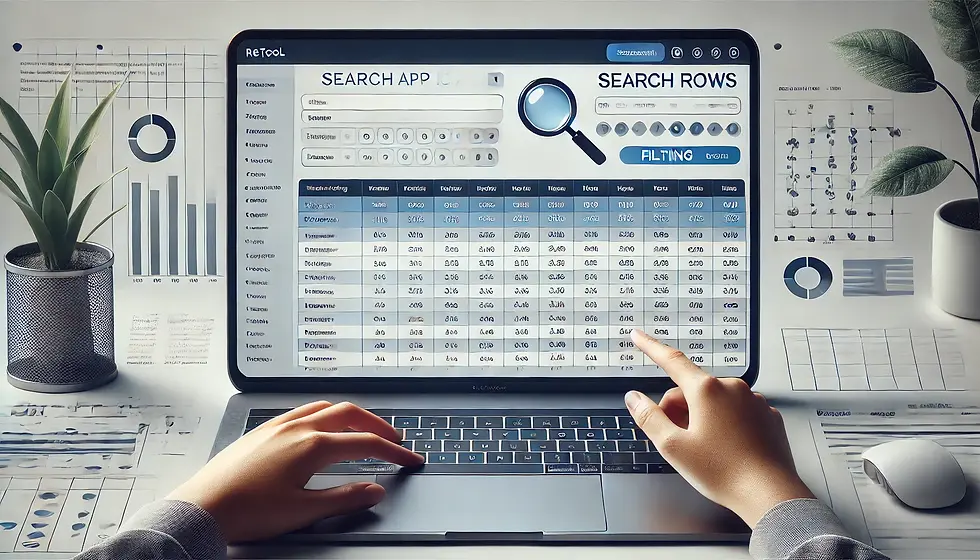
1. Effective Search Functionality
Ensuring that the search functionality is robust and intuitive, enabling users to filter through tables effortlessly.
User-Friendly Interface: Simple and intuitive design for entering search queries.
Real-Time Filtering: Immediate update of table data as the user types.
Highlighting Matches: Emphasizes search terms within the results for easy identification (by enabling Fuzzy match).
Advanced Search Options: Supports complex queries and multi-field searches.
Error Handling: Provides feedback for invalid inputs or no-result queries.
2. Challenges with Large Datasets
Addressing performance issues and ensuring that the search process is efficient, even when dealing with extensive amounts of data.
Performance Optimization: Strategies to handle large datasets without lag.
Pagination: Divides data into manageable chunks for display.
Server-Side Filtering: Offloads heavy processing to the server for very large datasets.
Data Caching: Caches frequently access data to speed up repeated searches.
Lazy Loading, which incrementally loads data as the user scrolls is something that Retool does not support and is a potential drawback.
3. Leveraging Frontend Filtering
Utilizing frontend filtering techniques to minimize server load and enhance performance, providing quick and responsive search results.
Client-Side Processing: Filters data directly in the browser for speed.
Asynchronous Updates: Uses asynchronous operations to keep the interface responsive.
Debouncing: Limits the frequency of queries to prevent overloading the system.
Efficient Data Structures: Implements optimized data structures for quick filtering.
Custom Filtering Logic: Tailors filtering logic to the application's specific needs.
4. User Experience Enhancement
Improving the overall user experience by providing a seamless and interactive search and filtering process.
Smooth Transitions: Ensures smooth visual transitions during filtering.
Consistent Performance: Maintains performance consistency, even with complex queries.
Interactive Feedback: Provides real-time feedback on search results and filters applied.
Mobile Optimization: Ensures search functionality works well on mobile devices.
Accessibility: Makes search features accessible to all users, including those with disabilities.
5. Integration and Maintenance
Ensuring the search functionality integrates well with other components and is easy to maintain.
Modular Design: Implements a modular approach for easy integration and maintenance.
Scalability: Ensures the search functionality can scale with growing data.
Documentation: Maintains comprehensive documentation for ease of use and maintenance.
Definition of Backend Filtering
Backend filtering involves processing search queries on the server side, using the server's resources to filter data based on user input.
In the context of Retool, backend filtering typically involves sending search parameters from a Retool application to a database server, which then performs the filtering operation using SQL queries or similar mechanisms. The filtered data is then sent back to the Retool application for display.
Role in Retool Search Input: Backend filtering enables powerful and efficient search functionality within Retool applications, especially when dealing with large datasets. By leveraging the server's processing power and database capabilities, backend filtering allows Retool applications to handle complex queries and large volumes of data that would be impractical to process entirely on the client side.
Common Approach Using SQL WHERE Clauses in Search Bars
A typical method for backend filtering is using SQL WHERE clauses in search queries. For example, to filter a table based on a search input:
SELECT * FROM table_A WHERE column_a iLIKE {{'%' + searchInput.value + '%'}}
This query searches for rows in table_A where column_a contains the value entered in the search bar.
Key Aspects of Backend Filtering in Retool
1. Query Construction:
Process: When a user inputs a search term in a Retool application, the input is used to construct a query that is sent to the backend database.
Example: For a search bar filtering records in a table, the query might look like
SELECT * FROM table_A WHERE column_a LIKE {{'%' + searchInput.value + '%'}}
2. Data Processing:
Server-Side Execution: The constructed query is executed on the server, leveraging the database's processing power to filter the data.
Efficiency: Backend databases are optimized for query processing, allowing for efficient handling of large datasets and complex filtering criteria.
3. Data Retrieval:
Result Set: After processing the query, the server returns the filtered dataset to the Retool application.
Display: The filtered data is then displayed in the relevant component (e.g., a table) within the Retool application, providing users with the search results.
4. Performance Considerations:
Scalability: Backend filtering helps manage large datasets by offloading processing from the client to the server.
Resource Management: Efficient backend filtering requires well-optimized queries and, potentially, database indexing to minimize performance lag.
5. Integration with Retool Components:
Dynamic Filtering: Retool components can dynamically update based on backend filtering results, ensuring that users always see the most relevant data.
Flexibility: Backend filtering allows for complex search functionalities, such as multi-field searches, sorting, and pagination, which can be seamlessly integrated into Retool applications.
Challenges Associated with Backend Filtering
1. Performance Lag with Large Datasets
Issue:
When dealing with large datasets (e.g., 5000+ records), backend filtering can result in significant performance lag.
Each search query requires a round-trip to the server, processing on the server, and then transferring the filtered data back to the client.
Impact:
Slow response times, leading to a poor user experience.
Increased load on the server, which can affect the performance of other operations and users.
2. High Server Load
Issue:
Frequent search queries from multiple users can overload the server.
Each query involves scanning the database, which can be resource-intensive, especially with complex filtering criteria.
Impact:
Potential server slowdowns or crashes.
Higher operational costs due to the need for a more robust server infrastructure.
3. Network Latency
Issue:
The time taken for data to travel between the client and server adds to the overall response time.
Network latency can be exacerbated by large data transfers, especially if the results are not paginated or limited.
Impact:
Users experience delays in receiving search results, particularly in geographically distributed applications.
Increased frustration for users, leading to decreased satisfaction and productivity.
4. Scalability Issues
Issue:
As the dataset grows, the time required to perform filtering operations increases.
Backend systems may struggle to scale efficiently with increasing data volumes and user queries.
Impact:
Degraded performance over time as data and usage scale.
Difficulty in maintaining acceptable response times without significant architectural changes.
5. Complexity in Query Optimization
Issue:
Optimizing SQL queries for performance can be complex and requires a deep understanding of the database schema and indexing.
Inefficient queries can exacerbate performance issues.
Impact:
Additional development and maintenance efforts to ensure queries are optimized.
There is a potential need for regular database tuning and reindexing.
You would have understood the backend filtering process in detail, so let's move on to the front-end filtering technique that will help you handle large data sets.
Frontend Filtering in Retool
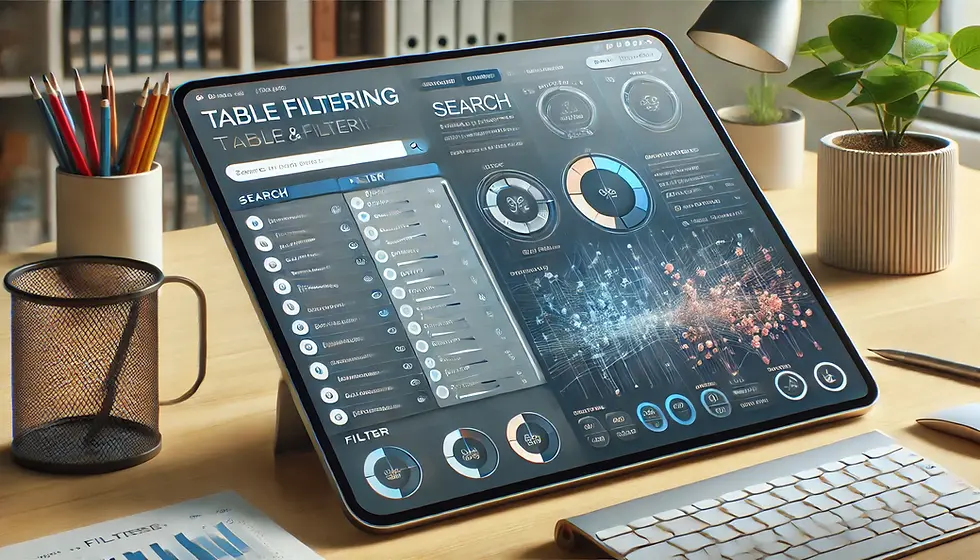
Frontend filtering involves processing search queries directly within the client-side application, using the browser's resources to filter data based on user input. In the context of Retool, frontend filtering means pulling data from a data source once and then applying filter criteria on the client side using JavaScript or other client-side technologies.
Role in Retool Search Input: Frontend filtering enhances user experience by providing quick and responsive search functionality. By performing the filtering operation on the client side, frontend filtering reduces the dependency on backend processing and network latency, allowing for instant updates to the displayed data as the user types in the search input.
Key Aspects of Frontend Filtering in Retool
1. Direct Data Manipulation:
Process: Data is initially pulled from the data source and stored on the client side. When a user inputs a search term, the filtering logic is applied directly to this data.
Example: Filtering a table of records based on the search input without sending a new query to the server.
2. Immediate Feedback:
Real-Time Updates: As users type in the search bar, the table updates instantly, providing immediate feedback without any delay caused by server communication.
Efficiency: This approach leverages the browser's processing power, making the filtering operation fast and seamless.
3. Reduced Server Load:
Minimized Requests: By handling filtering on the frontend, the number of requests sent to the server is reduced, lowering the load on the backend infrastructure.
Cost-Effective: This can save costs, especially for applications with high search activity and large user bases.
4. Enhanced User Experience:
Responsive Interface: Users experience a more interactive and responsive interface, as data filtering happens in real time.
Smooth Transitions: The absence of network delays ensures smooth transitions and more fluid user interaction.
5. Complex Filtering Logic:
Custom Filters: Frontend filtering allows for custom filtering logic tailored to specific application requirements.
Stacked Filters: Multiple filters can be applied sequentially, allowing users to refine their search results based on various criteria.
Steps for Implementing Frontend Filtering in Retool
Implementing frontend filtering in Retool involves setting up event handlers for the search bar to trigger filtering and using JavaScript to manipulate the filter stack for the table component. Here’s a step-by-step guide:
1. Leverage Event Handlers for the Search Bar to Trigger Filtering
Step 1: Add a Search Bar Component
Drag and drop a search bar component into your Retool application.
Step 2: Set Up an Event Handler
Configure an event handler for the search bar that triggers whenever the input value changes.
Use the onChange event to call a JavaScript query (defined in the next section) to handle the filtering logic.
2. Use JavaScript to Manipulate the Filter Stack for the Table Component
Step 1: Fetch and Store Data Locally
Use a query to fetch data from your datasource and store it in a local variable.
Example:
let allData = await dataSourceQuery.trigger();
Step 2: Define a Filter Function
Create a JavaScript query to filter the data based on the search term.
This query will manipulate the dataset stored locally and return the filtered results.
Example:
function filterTableData(searchTerm) {
const filteredData = allData.filter(row => {
// Adjust this condition based on the columns you want to search
return row.column_a.toLowerCase().includes(searchTerm.toLowerCase());
});
// Update the table with the filtered data
table.setData(filteredData);
}
Step 3: Update the Table Data
Use the table.setData method to update the table with the filtered data.
Example:
table.setData(filteredData);
With a solid grasp of backend and frontend filtering in Retool, you can implement efficient search functionalities that significantly enhance user experience.
Now, let's explore JavaScript event handlers, which are vital for making your applications interactive and responsive.
These handlers execute functions in response to user actions like typing in a search bar, clicking buttons, or selecting dropdown items, adding dynamic behavior to your web applications.
JavaScript Event Handlers in Retool
JavaScript event handlers are functions executed in response to specific events triggered by user interactions, such as typing in a search bar, clicking a button, or selecting an item from a dropdown. These handlers are essential for creating dynamic and interactive web applications.
Role in Retool Search Input: In Retool, JavaScript event handlers play a crucial role in managing user interactions with the search input component. They enable the application to respond immediately to user inputs by triggering appropriate actions, such as filtering data displayed in a table.
Steps for Setting Up JavaScript Event Handlers for Search Input
1. Setting Up Event Handlers for the Search Bar and Tie it to the Event Handler
Step 1: Add a Search Bar Component
Drag and drop a search bar component into your Retool workspace.
Step 2: Configure the Event Handler
Open the search bar's properties panel and locate the event handler section (e.g., onChange).
Step 3: Initialize Data and Store it
Ensure the initial data is fetched and stored
Example:
let allData = [];
// Fetch data and store it locally
async function initializeData() {
allData = await dataSourceQuery.trigger();
table.setData(allData);
}
Step 4: Write the JavaScript Filter Query for the Event Handler
Define the JavaScript query that will be executed when the event occurs.
Example:
function filterTableData(searchTerm) {
const filteredData = allData.filter(row => {
return row.column_a.toLowerCase().includes(searchTerm.toLowerCase());
});
table.setData(filteredData);
}
With a comprehensive understanding of JavaScript event handlers for filtering, the next step is to explore the various filter types and operators available in Retool.
Filter Types and Operators in Retool
Implementing effective search and filtering functionality in Retool involves understanding the different filter types and operators available. This allows you to customize how data is filtered and displayed based on user input.
1. Overview of Different Filter Types Available in Retool
Filter Types:
Text Filters: Filters data based on text input.
Example: Searching for names, descriptions, or any text field.
Numeric Filters: Filters data based on numeric values.
Example: Filtering prices, quantities, or any numeric field.
Date Filters: Filters data based on date values.
Example: Filtering records by creation date, last updated date, or any date field.
Boolean Filters: Filters data based on boolean values (true/false).
Example: Filtering records by status (active/inactive), completion (completed/not completed).
2. Common Operators
Operators:
1.includes: Checks if a field includes a specific value or substring.
Use Case: Searching for records where a name includes "John".
Example:
table.data.filter(row => row.name.includes('John'));
table.data.filter(row => ['active', 'pending'].includes(row.status));
2. equals: Checks if a field's value is equal to a specified value.
Use Case: Filtering records where the user ID equals 123.
Example:
table.data.filter(row => row.userId === 123);
Advanced Filtering Techniques in Retool
Implementing advanced filtering techniques can significantly enhance the search capabilities of your Retool applications. Here are three advanced techniques to consider:
1. Implementing Multiple Search Bars for Filtering on Different Columns
Step 1: Add Multiple Search Bars
You can drag and drop multiple search bar components into your Retool workspace, each corresponding to a different column you want to filter.
Step 2: Configure Event Handlers for Each Search Bar
Set up onChange event handlers for each search bar to capture user input and trigger the filtering logic.
Step 3: Write the Filtering Logic
Create a JavaScript function that reads values from all search bars and applies the filters accordingly.
2. Example JavaScript Code for Multi-Word Search and Filtering
Step 1: Write the Filtering Logic
Create a function that filters data based on multiple search terms. Each term must be present in at least one of the specified columns.
Tips and Best Practices for Efficient Filtering in Retool
Implementing efficient filtering in Retool requires careful planning and adherence to best practices to ensure optimal performance and user experience. Here are some key tips and best practices to follow:
1. Ensuring Efficient Frontend Filtering to Avoid Performance Issues
Best Practices:
Debouncing Input: Implement debouncing on search inputs to prevent excessive re-rendering and filtering operations. This technique delays the filtering action until the user has stopped typing for a specified period.
Optimized Data Structures: Use efficient data structures and algorithms for filtering. For example, converting strings to lowercase once before filtering to avoid repeated conversions.
Lazy Loading: Implement lazy loading or pagination to manage large datasets, ensuring that only a subset of data is processed at a time. (Lazy loading is not possible on Retool. However, server side pagination can be used)
Client-Side Caching: Cache data on the client side to reduce redundant data fetching and improve performance.
2. Testing and Validating Filtering Logic with Sample Datasets
Best Practices:
Sample Data: Use representative sample datasets to test and validate your filtering logic. This helps identify potential issues and ensures the logic works correctly with real-world data.
Unit Testing: Write unit tests for your filtering functions to ensure they handle various input scenarios correctly.
Performance Testing: Measure the performance of your filtering logic with large datasets to ensure it scales effectively and remains responsive.
Conclusion
Implementing effective search and filtering in Retool can significantly enhance user experience, especially when dealing with large datasets. By leveraging both backend and frontend filtering techniques, you can ensure responsive and accurate search results.
Toolpioneers is here to help you maximize Retool's capabilities, offering expert guidance and support to streamline your application development process. Whether you need assistance with search functionalities or other Retool components, our team is ready to assist.
Ready to optimize your Retool applications? Contact Toolpioneers today for comprehensive support and expert solutions.