Continuous Integration (CI) is a software development practice where developers frequently integrate their code changes into a shared repository, often multiple times daily.
The process is made efficient and speedy by automated builds and tests that automatically verify each integration to detect integration errors as quickly as possible. The primary purpose of CI is to prevent integration problems, improve software quality, and reduce the time taken to deliver new software updates.
This article will help you understand the importance and benefits of continuous integration in your projects.
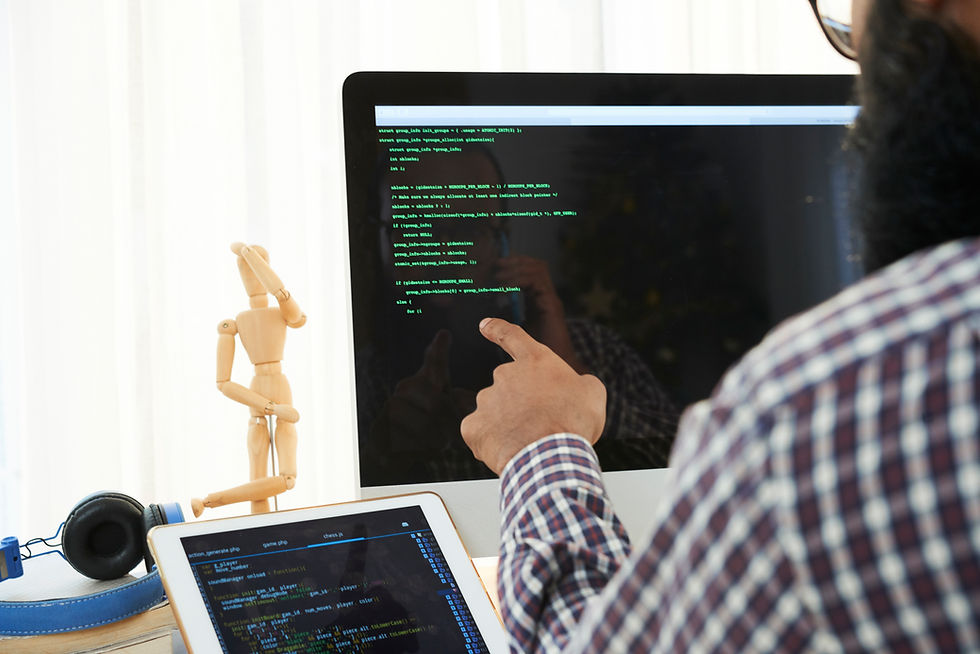
Importance of CI in Facilitating Agile Development and Maintaining a Stable Codebase
CI is crucial for Agile development methodologies because it supports frequent iterations and continuous feedback. By integrating code changes regularly, CI helps in:
Early Detection of Issues: Identifying and fixing integration issues early in the development cycle.
Stable Codebase: Maintaining a stable and functional codebase throughout the development process.
Faster Feedback Loop: Providing immediate feedback to developers, allowing them to address issues quickly.
Enhanced Collaboration: Encouraging collaboration among team members by integrating changes frequently
Key Steps in Developing and Integrating Code with CI
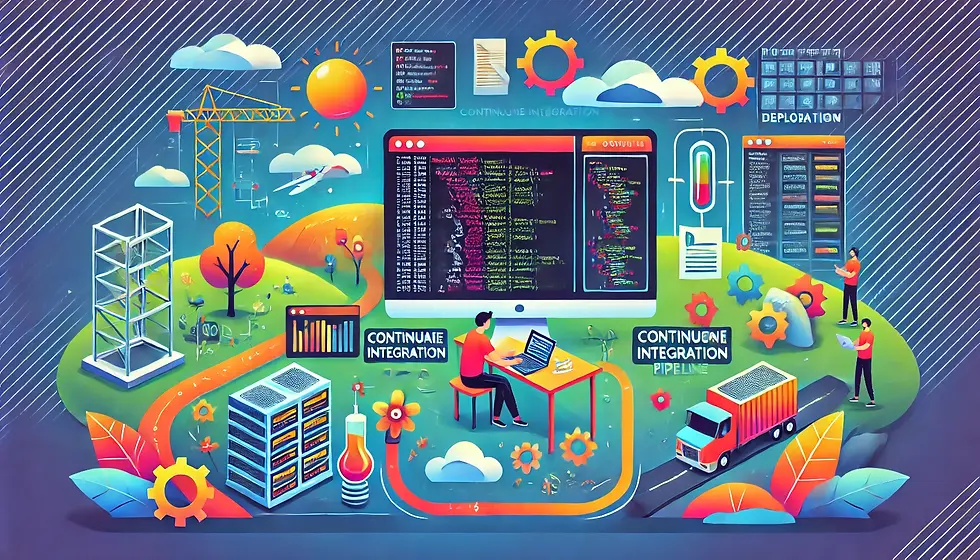
1. Developing Features in Small, Manageable Iterations
Incremental Development: Break down features into small, manageable parts that can be developed and integrated independently.
Frequent Integrations: Integrate code changes frequently to avoid large, complex merges and reduce integration issues.
Continuous Feedback: Provide continuous feedback to developers, allowing them to address issues early in the development cycle.
2. Setting Up Automated Testing
Unit Tests: Write unit tests for individual components to ensure they work correctly in isolation.
Integration Tests: Develop integration tests to verify that different system components work together as expected.
Automated Test Execution: Configure the CI system to automatically execute these tests whenever code changes are committed to the repository.
3. Using Version Control Systems Effectively
Version Control System (VCS): Use a robust VCS like Git to manage code changes and track the history of modifications.
Committing Changes: To keep the repository up to date, commit code changes frequently with clear, descriptive commit messages.
Pull Requests: Use pull requests to review and discuss code changes before merging them into the main branch. This ensures that code quality and standards are maintained.
Branching Strategy: Implement an effective branching strategy (e.g., GitFlow) to manage feature development, hotfixes, and releases.
4. Implementing Integration Approval Steps
Automated Tests: Set up the CI system to run automated tests on each commit or pull request to catch issues early.
Code Quality Checks: Use static code analysis tools to check for code quality issues, such as code smells, security vulnerabilities, and potential bugs.
Syntax Style Reviews: Enforce coding standards and style guidelines using tools like ESLint or Prettier to automatically review code syntax and style.
Manual Code Reviews: Conduct manual code reviews to ensure code quality, maintainability, and adherence to design principles.
By following these steps, teams can ensure that code is developed and integrated smoothly with continuous integration practices, leading to higher code quality, faster feedback cycles, and a more efficient development process.
Getting Started with Continuous Integration
1. Necessity of a Version Control System and Choosing a Hosting Platform
Version Control System (VCS):
Definition: A version control system tracks changes to source code, enabling multiple developers to collaborate on the same project seamlessly.
Popular VCS Tools: Git, Mercurial, Subversion.
Key Benefits:
Keeps track of code history and allows for reverting changes.
Facilitates collaboration among team members.
Provides a centralized location for the codebase.
Choosing a Hosting Platform:
Popular Hosting Platforms: GitHub, GitLab, Bitbucket.
Factors to Consider:
Integration with CI Tools: Ensure the platform supports integration with CI tools.
Access Control: Provides robust access control mechanisms to secure your codebase.
Cost: Consider the pricing plans and choose one that fits your budget.
Community and Support: Opt for platforms with strong community support and documentation.
2. Adopting CI in Five Steps
Set Up a Version Control System (VCS):
Create a Repository: Initialize a repository on your chosen hosting platform.
Branching Strategy: Define a branching strategy to manage different code versions.
Example:
Initialize a Git repository: git init
Create a new branch: git checkout -b develop
Automated Testing:
Write Tests: Develop unit, integration, and end-to-end tests for your codebase.
Configure Testing Frameworks: Set up frameworks like JUnit, Pytest, or Jest.
Automate Tests: Use CI tools to run tests automatically on every code push.
3. Integrate CI Tools:
Choose a CI Tool: Select a CI tool like Jenkins, Travis CI, or CircleCI.
Configure CI Pipeline: Create a pipeline to automate building, testing, and deploying your code.
4. Code Quality Checks:
Static Analysis: Implement static code analysis tools like ESLint or SonarQube.
Automate Quality Checks: Integrate these tools into your CI pipeline to ensure code quality.
5. Integration Tutorials and Documentation:
Create Tutorials: Develop guides and tutorials for team members to understand the CI setup.
Document Processes: Maintain comprehensive documentation for all CI processes and configurations.
Example:
Create a README.md with instructions on running and testing the code locally and in the CI pipeline.
3. Benefits of Integrating CI for Different Organizational Departments Beyond Development
Development:
Early Bug Detection: CI helps identify and fix bugs early in development.
Code Quality: Maintains high code quality through automated testing and static analysis.
Operations:
Deployment Efficiency: CI pipelines automate the deployment process, reducing manual intervention and errors.
Infrastructure Management: Simplifies infrastructure management by integrating with tools like Docker and Kubernetes.
Quality Assurance:
Consistent Testing: Ensures consistent and thorough testing for every code change.
Reduced Regression: Automated tests help catch regressions early, maintaining the application's stability.
Product Management:
Faster Release Cycles: CI enables more frequent and reliable releases, improving time-to-market.
Improved Visibility: Provides better visibility into the development process through dashboards and reports.
Business:
Cost Efficiency: Reduces costs associated with manual testing and deployment.
Customer Satisfaction: Delivers higher quality products to customers, enhancing satisfaction and trust.
By implementing CI, organizations can streamline their development workflows, improve code quality, and enhance collaboration across various departments.
This ultimately leads to better software products and increased operational efficiency. The next step is to learn how to implement continuous integration successfully in your project workflow.
Implementing Continuous Integration Workflow
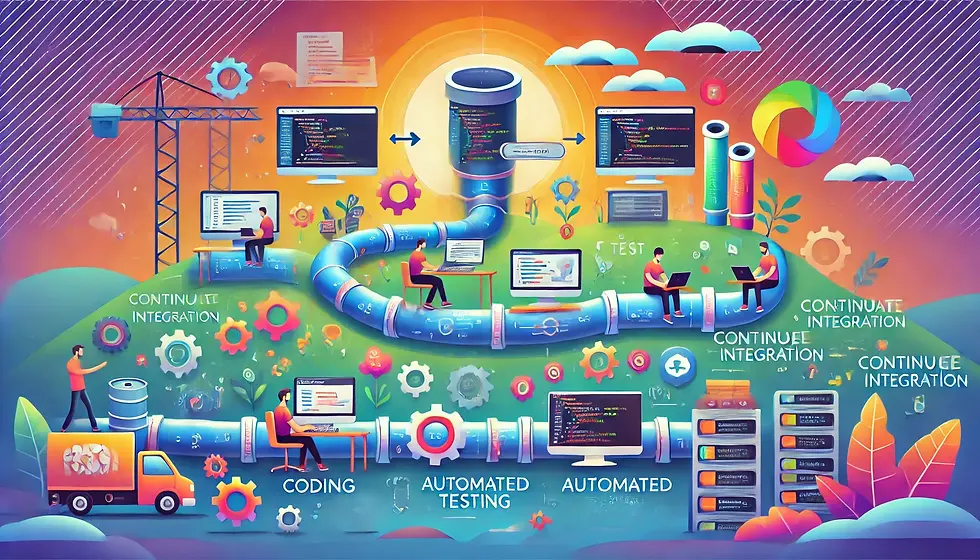
1. Overview of a Typical CI Workflow
Cloning the Repository: Start by cloning the main code repository to your local development environment.
Feature Development: Develop new features or make improvements in a local branch.
Code Analysis and Automated Testing: Run automated tests and static code analysis tools to identify and fix errors early.
Committing and Pushing Changes: Commit changes to the local branch and push them to the remote repository.
Continuous Integration Platform: The CI platform detects changes and automatically runs a series of pre-configured tasks.
Feedback Loop: Developers receive immediate feedback on build and test results, enabling quick error resolution and reintegration.
2. Phase 1: Developing and Qualifying Features in the Local Repository
Cloning the Repository:
Use the git clone command to copy the repository to your local machine.
Example: git clone https://github.com/your-repo/project.git
Creating a Branch:
Create a new branch for your feature development.
Example: git checkout -b feature/new-feature
Developing Features:
Write code and develop features in your local branch.
Running Local Tests:
Run unit tests and integration tests locally to ensure the new code doesn't break existing functionality.
Committing Changes:
Commit your changes with descriptive messages.
Example: git commit -m "Add new feature for user authentication"
Pushing Changes:
Push the changes to the remote repository.
Example: git push origin feature/new-feature
3. Phase 2: Execution of Automated Tasks on a CI Platform
CI Platform Setup:
Configure a CI platform like Jenkins, CircleCI, or Travis CI to monitor the repository for changes.
Running Pipelines:
Set up pipelines to automatically run tasks such as compiling code, running tests, and analyzing code quality.
Example: Configure a Jenkins pipeline to run on every push to the repository.
Pipeline Execution:
The CI platform triggers the pipeline when changes are pushed to the repository.
Automated tasks such as build, test, and deploy are executed.
Results and Feedback:
The CI platform provides immediate feedback on the status of the build and tests.
Developers receive notifications of success or failure, including detailed logs and reports.
4. Phase 3: Investigating and Resolving Failures
Identifying Failures:
Review the feedback from the CI platform to identify any build or test failures.
Example: Jenkins console output shows a test failure due to a syntax error.
Diagnosing Issues:
Analyze logs and error messages to diagnose the root cause of the failures.
Example: Test logs indicate a null pointer exception in the authentication module.
Fixing Issues:
Make necessary code changes to resolve the identified issues.
Example: Add null checks and re-run local tests to confirm the fix.
Re-triggering the Pipeline:
Commit and push the fixed code to the repository.
The CI platform automatically re-triggers the pipeline to verify the fix.
Example: git commit -m "Fix null pointer exception in authentication module"
Ensuring Successful Integration:
Ensure all automated tasks pass successfully, confirming that the new changes integrate well with the existing codebase.
By following these phases and utilizing a robust CI workflow, developers can ensure smooth integration of new features, maintain code quality, and quickly address any issues that arise during development.
Benefits of Continuous Integration
1. Improvement in Coordination and Communication Within Teams
Seamless Collaboration: CI tools and practices ensure all team members work with the latest codebase, minimizing conflicts and integration issues.
Transparency: Regular integration of code changes allows team members to see each other's work, fostering better collaboration and understanding.
Standardization: CI establishes consistent processes and standards for code integration, enhancing team coordination.
2. Enhanced Feedback Loops for Quicker Debugging and Issue Resolution
Immediate Feedback: Automated testing and integration provide immediate feedback on code changes, enabling developers to identify and fix issues quickly.
Early Detection of Bugs: Continuous testing catches bugs early in the development cycle, reducing the time and effort needed for debugging.
Faster Iterations: Quick feedback loops allow for faster iterations and continuous improvement of the codebase.
3. Organizational Benefits Such as Scalability, Reduced Overheads, and Improved State of Software Communication
Scalability: CI systems can easily scale with the project's growth, handling increased code changes and integrations without compromising performance.
Cost Reduction: Automating repetitive tasks reduces the need for manual intervention, lowering operational costs and freeing up resources for more critical tasks.
Efficient Resource Management: CI practices optimize resource allocation, ensuring teams work efficiently and effectively.
4. Increased Software Quality and Reliability
Consistent Testing: Regular automated testing ensures that the codebase remains stable and reliable, reducing the risk of defects in production.
Code Quality Assurance: Continuous code quality checks and static analysis tools help maintain high code standards, resulting in robust and maintainable software.
Confidence in Releases: CI practices provide confidence in the software's quality, enabling more frequent and reliable releases.
5. Enhanced Deployment and Delivery Processes
Automated Deployment: CI tools often integrate with continuous deployment (CD) systems, automating the deployment process and reducing manual errors.
Faster Time-to-Market: By streamlining development, testing, and deployment processes, CI helps deliver features and updates to the market faster.
Continuous Delivery Pipeline: CI supports the creation of a continuous delivery pipeline, ensuring that software is always deployable.
Challenges and Best Practices in Continuous Integration
Continuous Integration (CI) is a cornerstone of modern software development, enhancing productivity and code quality. However, implementing CI comes with its own set of challenges. Understanding these challenges and adhering to best practices is crucial for successful CI adoption.
Understanding the Organizational and Technical Challenges in Adopting CI
Organizational Challenges:
Cultural Shift: Transitioning to CI requires a cultural change where teams embrace frequent integration and automated testing.
Skill Gaps: Ensuring all team members are proficient with CI tools and practices can be a hurdle.
Resource Allocation: Initial setup and ongoing maintenance of CI systems can demand significant resources.
Technical Challenges:
Tool Integration: Integrating CI tools with existing development environments and workflows can be complex.
Infrastructure: Setting up and maintaining the infrastructure required to support continuous integration can be resource-intensive.
Testing Challenges: It can be difficult to develop comprehensive test suites that run efficiently and cover all critical aspects of the application.
Best Practices for a Successful CI Implementation
Test-Driven Development (TDD):
Write Tests First: Develop tests before writing the actual code to ensure that the code meets the required functionality.
Frequent Testing: Run automated tests frequently to catch issues early and ensure code quality.
Pull Requests and Code Review:
Code Reviews: Implement mandatory code reviews for all pull requests to maintain code quality and share knowledge within the team.
Collaborative Reviews: Encourage collaborative reviews to foster a culture of continuous learning and improvement.
Optimizing Pipeline Speed:
Parallel Testing: Run tests in parallel to reduce the overall time taken for the CI pipeline.
Incremental Builds: Use incremental builds to recompile and test only the changed components, speeding up the process.
Caching: Implement caching mechanisms for dependencies and intermediate build artifacts to avoid redundant processing.
Scaling Up Engineering Team Output:
Automation: Automate as many processes as possible, including testing, deployment, and code quality checks, to allow developers to focus on writing code.
Modular Development: Encourage modular development practices to facilitate the management and integration of code changes from different team members.
Working Alongside Agile Development Workflows:
Frequent Integrations: Align CI practices with agile methodologies by integrating code frequently, ideally multiple times a day.
Sprint Planning: Incorporate CI considerations into sprint planning to ensure all planned work includes integration and testing tasks.
Retrospectives: Use agile retrospectives to review CI processes and identify areas for improvement.
By understanding the challenges and adopting these best practices, organizations can successfully implement Continuous Integration, leading to more efficient development processes, higher code quality, and improved collaboration among development teams.
Conclusion
Continuous Integration (CI) has become a cornerstone of modern, efficient, high-output software development organizations. By enabling frequent code integrations, automated testing, and immediate feedback, CI helps maintain a stable codebase, enhances team collaboration, and accelerates the development lifecycle.
Adopting CI practices is crucial for organizations aiming to improve their software development processes, achieve higher-quality outputs, and remain competitive in a rapidly evolving technological landscape.
You can opt for platforms like Retool for your software development process that can ultimately support continuous integration and help you reap the benefits that will contribute to the success of your project.
Leverage the full capabilities of your software development process with Retool and ToolPioneer's expertise. From streamlining system development and enhancing team collaboration to implementing robust CI/CD practices, Retool empowers you to achieve your development goals.