Guide to Using Temporary States and Variables in Retool
- Yashaswi Raghuveer Meduri
- Oct 8, 2024
- 10 min read
Updated: Dec 13, 2024
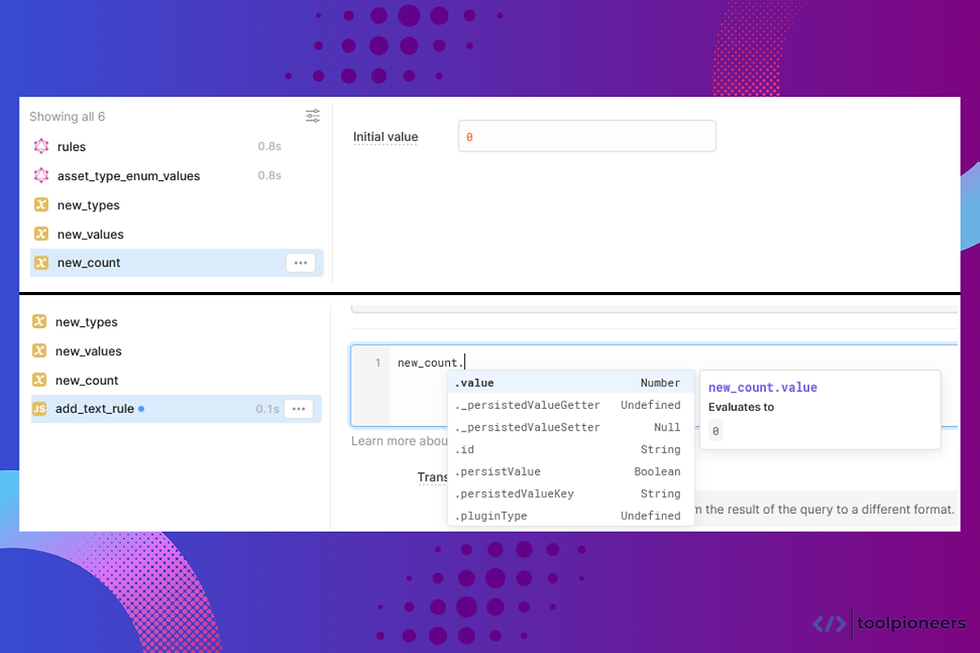
Retool state variables, also known as temporary states and variables, are practical tools that empower users to manage and manipulate data dynamically within their applications.
These tools provide a flexible and efficient way to handle data, perform calculations, and control the flow of information. By doing so, they make your Retool applications more efficient, saving time and resources.
This comprehensive guide will delve into Retool state variables, their purposes, and how they can enhance the functionality of your Retool projects. Understanding and effectively utilizing these features can significantly improve your application's performance and user experience.
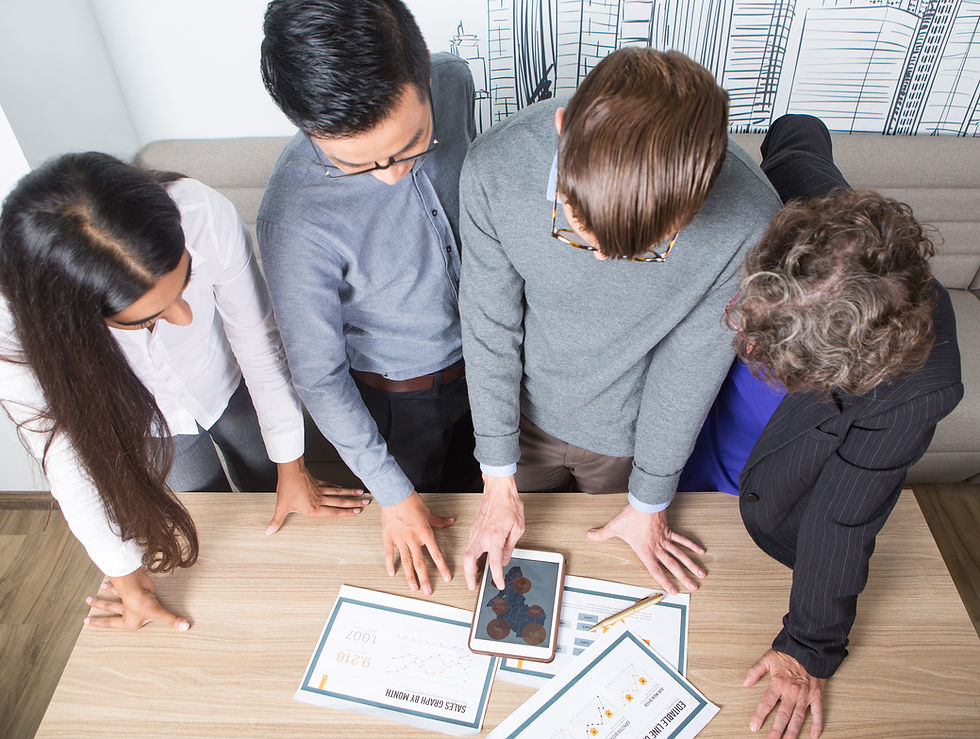
Definition of Retool State Variables
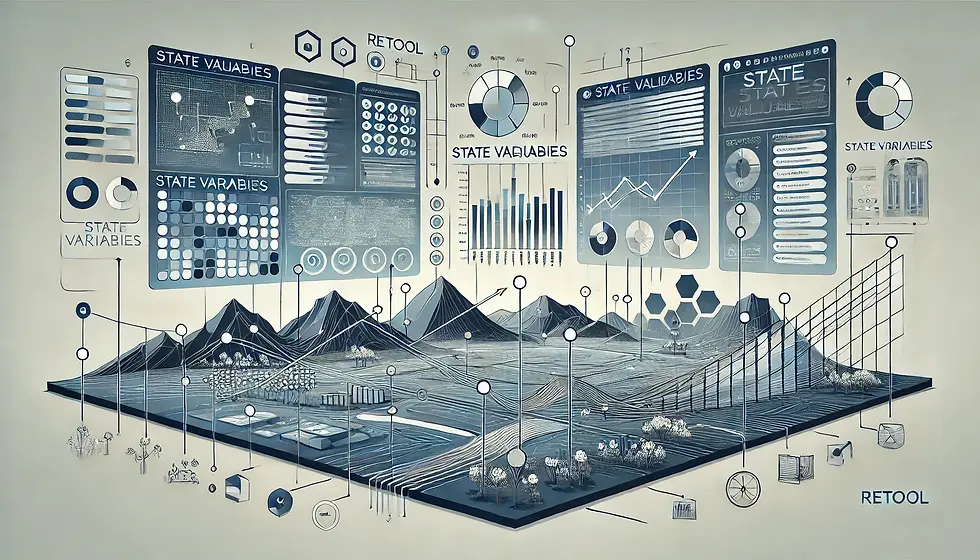
Retool State Variables are dynamic storage units within Retool applications that allow developers to manage and manipulate data temporarily during an application session. These variables store values such as user inputs, API responses, or intermediate computation results, enabling real-time updates and interactions without needing to refresh or reload the entire application.
Key Characteristics of Retool State Variables:
Dynamic and Temporary: Retool state variables exist only for the duration of the session. They handle data that needs to change frequently and respond to user interactions in real-time.
Session-specific: These variables store data specific to the current user session and do not persist beyond it. Once the session ends, the data is discarded.
Interactive: They facilitate dynamic interactions within the application, allowing components to react immediately to changes in data. For example, they can dynamically update a chart based on user input or filter a data table.
Efficient Data Handling: By storing intermediate results and frequently changing data locally, state variables reduce the need for repeated database queries or API calls, enhancing the application's performance.
Conditional Logic and State Management: Retool state variables are essential for managing the application state and implementing conditional logic. They enable developers to control the visibility of components, trigger workflows, and perform calculations based on their values.
Example Use Cases:
User Input Storage: Temporarily store user inputs before submitting them to a backend service or API.
Intermediate Calculations: Hold intermediate results from calculations or data transformations needed for subsequent steps.
UI State Management: Manage the visibility and state of UI components, such as showing or hiding sections based on user interactions.
Variables in Retool
Variables in Retool are essential for managing data within your application. They provide a flexible way to temporarily store and manipulate data, supporting simple and complex operations.
Features and Benefits:
Temporary Data Storage:
Functionality: Variables allow you to temporarily hold data, including user inputs, intermediate computation results, or data fetched from external sources, during a session.
Benefit: This ensures that data is readily available within the session, enhancing responsiveness and interactivity.
Also read: Quickstart for Retool Database
Session-specific Persistence:
Functionality: Variables are only available during the current session and do not persist once the app is reloaded or closed.
Benefit: Provides a clean slate for each new session, ensuring that old data does not interfere with new operations.
Read and Write Access Anywhere in the App:
Functionality: Retool variables can be accessed and modified at any point within the application.
Benefit: This versatility allows different app components to share and update the same data, ensuring consistency and reducing redundant data fetching or computation.
Automatic Reset on App Reload:
Functionality: Variables reset every time the app is loaded.
Benefit: This feature is handy for temporarily retaining user inputs and performing complex logic without manual cleanup.
Support for Complex Logic and Data Transformation:
Functionality: Variables can hold intermediate results for complex calculations or data transformations that must be accessed across different app parts.
Benefit: Facilitates the implementation of advanced functionality and workflows, making it easier to manage and manipulate data dynamically within the app.
Example Use Cases and Scenarios
Dynamic Form Handling:
Use Case: Storing user inputs temporarily as they fill out a multi-step form.
Benefit: Ensures users can navigate between form steps without losing their inputs, enhancing the overall user experience.
Real-time Data Visualization:
Use Case: Updating charts and graphs based on user-selected filters or parameters.
Benefit: Allows real-time data visualization without repeated server calls, making the application more responsive.
Conditional UI Rendering:
Use Case: Showing or hiding sections of the application based on user roles or permissions.
Benefit: Ensures that users only see the parts of the application that are relevant to them, improving usability and security.
Intermediate Computation Storage:
Use Case: Storing intermediate results of complex calculations used in multiple places within the app.
Benefit: Reduces redundant computations, leading to more efficient and maintainable code.
Session-based User Experience:
Use Case: Retaining user settings or preferences for the duration of a session.
Benefit: Enhances personalization by temporarily remembering user choices and configurations, improving the overall user experience.
By leveraging the benefits of Retool state variables, you can create more dynamic, efficient, and user-friendly applications.
Creating and Managing Variables in Retool
Creating and managing variables in Retool is a straightforward process that enables you to store and manipulate data dynamically within your applications. Follow these steps to create and manage variables effectively:
Creating Variables
Open the Code List:
Navigate to the "Code" section in your Retool application.
This section allows you to manage scripts, queries, and variables used within your app.
Create Variables:
Click the "+" button to add a new variable.
Assign a name to your variable to identify it quickly.
Optionally, set an initial value if needed.
Managing Variable Values
Once you have created variables, you can manage their values using setValue and setIn. These methods allow you to update the values stored in your variables dynamically.
setValue Method:
Functionality: This method is used to set or update the value of a variable.
Usage:
variableName.setValue(newValue);
Example:
userName.setValue("John Doe");
Benefit: Directly updates the variable's value, making it immediately available for other components and scripts in the app.
setIn Method:
Functionality: This method sets or updates a nested value within a variable.
Usage:
variableName.setIn(["nestedKey"], newValue);
Example
userDetails.setIn(["address", "city"], "New York");
Benefit: Allows for precise updates within nested data structures, ensuring that only the intended part of the variable is modified.
Example Use Case
Consider a scenario where you want to manage user input dynamically within your app:
Create a Variable:
Open the Code list.
Click the "+" button to create a variable named userInput.
Optionally, set an initial value like "" (an empty string).
Update the Variable:
Use the setValue method to update userInput based on user interactions.
For example, when a user types into an input field:
userInput.setValue(inputField.value);
3. Nested Data Management:
If userInput contains nested data, use setIn to update specific parts.
For example, updating the user's city:
userInput.setIn(["address", "city"], "San Francisco");
By following these steps, you can effectively create and manage variables in Retool, ensuring that your application remains dynamic, responsive, and capable of handling complex data interactions.
Now, learn how to use JavaScript to manage the created variables.
Benefits of Retool State Variables
1. Enhanced Responsiveness and Interactivity:
Description: Retool state variables allow your application to react instantly to user inputs and interactions without needing to refresh the entire app.
Benefit: Provides a smoother and more engaging user experience by ensuring that changes are immediately reflected in the application.
2. Simplified Data Management:
Description: These variables enable you to store and manage data locally within the app session, including user inputs, intermediate results, and fetched data.
Benefit: Reduces the complexity of handling data, making it easier to perform calculations, transformations, and conditional logic within your application.
3. Improved Performance:
Description: Retool state variables minimize the need for repeated database queries or API calls by storing frequently accessed and dynamically changing data locally.
Benefit: Enhances the performance of your application, leading to faster load times and a more responsive interface.
4. Flexibility in Application Logic:
Description: State variables support complex application logic, such as conditionally showing or hiding components, triggering workflows, and updating UI elements based on variable values.
Benefit: Provides more significant control over the application’s behavior, allowing for more sophisticated and tailored user experiences.
5. Temporary and Session-specific Data Handling:
Description: State variables reset with each new session, ensuring that data from previous sessions does not interfere with current operations.
Benefit: Provides a clean slate for each user session, which is helpful for applications that require temporary data storage and ensures consistency and accuracy in data handling.
Common Issues with Temporary States in Retool
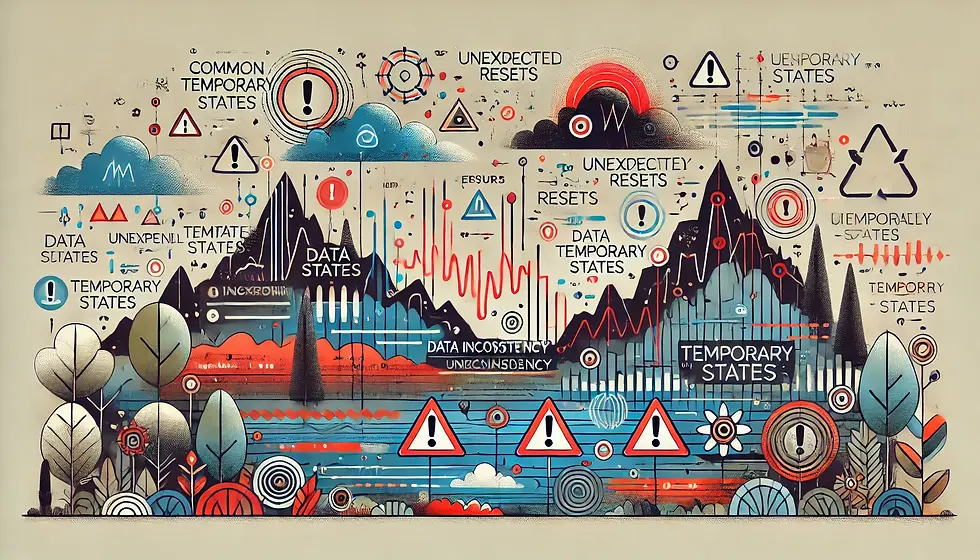
1. Issues Faced When Not Updating State Values in Loops and Triggering Resource Queries
Description:
When using temporary states within loops, especially in scenarios where these states are meant to trigger resource queries, you may encounter issues if the state values need to be updated correctly.
Common problems include infinite loops, failure to trigger queries or inconsistent data states.
Problem Scenarios:
Infinite Loops: Failing to manage state updates in loops properly can result in continuous triggering of the same state update, leading to an infinite loop.
Query Failures: If state values are not updated as expected within loops, resource queries dependent on these state values may not trigger, leading to data retrieval issues or incomplete operations.
Inconsistent Data States: With proper state management, the application may reflect consistent data states, causing confusion and potential errors in the application logic.
Solutions:
Use Debouncing: Implement debouncing techniques to ensure state updates occur at controlled intervals, preventing rapid and repetitive state changes.
Conditional Updates: Add conditions to state updates within loops to ensure they only occur when necessary, avoiding redundant operations.
Monitor State Changes: Use event listeners or watchers to monitor state changes and ensure resource queries are triggered correctly based on updated state values.
2. Temporary State Reset and Handling Null Values
Description:
Temporary states in Retool reset with each new session can lead to issues if the application logic does not account for this reset behavior.
Handling null or undefined values in temporary states is crucial to avoid errors and ensure smooth application performance.
Problem Scenarios:
State Reset: When a session ends, all temporary states reset. If the application relies on these states without reinitializing them, it can lead to errors or unexpected behavior.
Null Values: Temporary states may sometimes hold null or undefined values, leading to errors in computations, UI rendering, or data processing.
Solutions:
Initialize States: Always initialize temporary states with default values to ensure the application has a known starting point.
Check for Null Values: Implement null checks and default value assignments in your application logic to handle cases where temporary states might be null or undefined.
Session Persistence: For critical state data that should persist across sessions, consider using more permanent storage solutions, such as local storage or database entries, instead of relying solely on temporary states.
Solutions and Best Practices for Temporary States in Retool
To manage temporary states effectively in Retool, you must avoid common pitfalls like race conditions and ensure you handle state updates correctly. Here are some solutions and best practices:
1. Avoiding Race Conditions and Possible Software Version Discrepancies
Race Conditions:
Problem: Race conditions occur when multiple operations attempt to update the state simultaneously, leading to unpredictable behavior and potential data inconsistency.
Solution: Use synchronization mechanisms to ensure that state updates occur in a controlled manner.
Software Version Discrepancies:
Problem: Different versions of the software running concurrently might handle state updates differently, causing inconsistencies.
Solution: Implement version control and update strategies to ensure all application components use compatible versions.
Best Practices:
Atomic Operations: Ensure state updates are atomic, meaning they are complete as a single, indivisible operation.
Locks and Mutexes: Use locking mechanisms or mutexes to prevent multiple operations from updating the state simultaneously.
Version Checks: Implement version checks to ensure that state updates are compatible with the current version of the application.
Centralized State Management: Centralize state management to reduce the chances of conflicting updates from different application parts.
2. Using await for State Updates
Problem: When updating states asynchronously, failing to use await can cause state updates to occur out of order, causing inconsistent states.
Solution: Always use await for state updates to ensure each update completes before the next one begins.
Best Practices:
Async/Await: Use async/await to manage asynchronous operations, ensuring that state updates are handled in the correct order.
Chaining Promises: Chain promises to ensure they execute sequentially for complex state updates involving multiple asynchronous operations.
Advanced Usage and Workarounds for Temporary States in Retool
In Retool, advanced techniques and workarounds can help you manage temporary states more effectively and avoid common pitfalls. Here are some strategies to enhance your application's performance and reliability:
1. Capturing a Reference to Avoid Quick Changes
Problem: Quick, successive changes to a temporary state can lead to unpredictable behavior or performance issues.
Solution: Capture a reference to the state value before making changes, ensuring your logic operates on a stable state snapshot.
Best Practices:
Snapshot State Values: Capture the current state value in a variable before making changes to ensure consistency.
Debouncing: Implement debouncing techniques to limit the frequency of state updates.
2. Passing Values Directly to Queries Without State Updates
Problem: Updating the state to pass values to queries can be inefficient and lead to unnecessary state changes.
Solution: Pass values directly to queries without updating the state, reducing overhead and improving performance.
Best Practices:
Direct Query Parameters: Use query parameters to pass values directly to your queries.
Avoid Redundant State Updates: Skip state updates when the sole purpose is to pass data to a query.
By implementing these advanced usage techniques and workarounds, you can enhance your Retool applications, ensuring they are both performant and reliable.
How Toolpioneers Can Help You?
Toolpioneers is dedicated to enhancing your Retool experience by offering expert guidance and tailored solutions. Our team of professionals can assist you in effectively utilizing Retool state variables to create dynamic, responsive, and efficient applications.
Whether you need help with setting up your state management, optimizing data handling, or implementing complex workflows, Toolpioneers provides the expertise to ensure your Retool projects are successful. With our support, you can focus on innovation and performance, leaving the technical complexities to us.
Partner with Toolpioneers to elevate your Retool applications and achieve your business goals seamlessly.
Frequently Asked Questions
1. Can state variables in Retool persist data across sessions?
No, state variables in Retool are session-specific and do not persist data across sessions. Once the session ends or the app is reloaded, the data stored in state variables is discarded. For data persistence across sessions, consider using more permanent storage solutions like databases or local storage.
2. Are there any performance considerations when using state variables in Retool?
Yes, using state variables can significantly improve the performance of your Retool application by reducing the need for repeated database queries or API calls. However, it’s essential to manage them efficiently to avoid issues like unnecessary state updates or race conditions, which can affect performance.
3. Can I use state variables in combination with other data sources in Retool?
Yes, you can use state variables alongside other data sources like APIs, databases, and third-party services. State variables can store intermediate results, user inputs, or data transformations that complement the data fetched from other sources, enhancing your app’s functionality.
4. How do I debug issues with state variables in Retool?
To debug issues with state variables, you can use console logs to track the values of variables at different points in your application. Additionally, Retool provides a debug mode where you can inspect variable values and see how they change in real-time. This helps identify and resolve issues related to state management.